How to create a random string in PHP

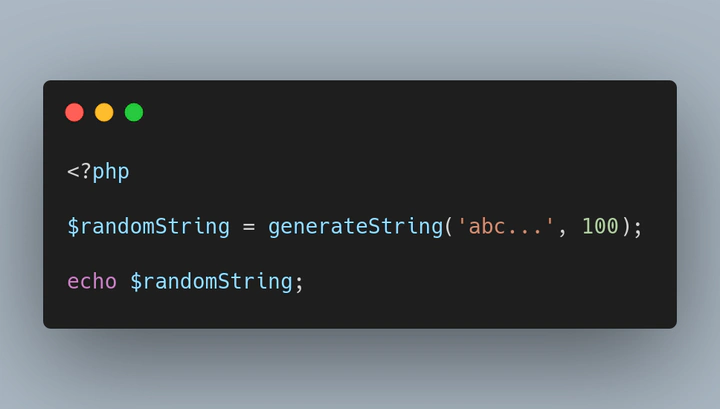
There are a lot of ways to generate a random string in PHP. In this post we’ll take a look at two options we have, and how to use them.
The Randomizer
The Randomizer class (introduced in PHP 8.2) is arguably the best way in OOP PHP to use randomness, and should be used whenever possible.
It comes with 2 methods for creating random strings. Where getBytes
will give you a random set of bytes of the specified length, but
characters may not be printable, or valid UTF-8. The second one, is the one you’ll likely use most of the time, which allows you to specify what
characters you want in your output string. If you specify a character multiple times in the $string
variable, it is more likely to occur.
// Introduced in PHP 8.2
public getBytes(int $length): string
// Introduced in PHP 8.3
public getBytesFromString(string $string, int $length): string
How to use the Randomizer
The randomizer class takes an Engine
interface as an optional argument. If not provided, PHP will use the Secure
variant.
So lets write a class that generates a random subdomain for a website.
use Random\Randomizer;
class SubDomainBuilder
{
private const KEY_SPACE = 'abcdefghijklmnopqrstuvwxyz0123456789';
public function __construct(
private Randomizer $randomizer,
) {}
public function createsubDomain(string $domain): string
{
return sprintf(
'%s.%s',
$this->randomizer->getBytesFromString(self::KEY_SPACE, 8),
$domain
);
}
}
$builder = new SubDomainBuilder(new Randomizer());
echo $builder->createsubDomain('example.com') . "\n";
In this SubDomainBuilder
class, we have the createsubDomain
method, where we use the getBytesFromString
method of the Ranomizer
.
We pass it a keyspace of a through z, and the numbers 0 to 9, so we get a sub domain which is only lowercased letters and numbers.
Running this script will output something simmilar to x0elme6k.example.com
.
One of the great things about the Randomizer
class is that you can provide an engine, and can seed that engine. This will make the
results deterministic, and allow you to use the randomness in unit tests.
For example, if we change the using of the class to the following, we’ll get the same results every single time. (nleajcf2.example.com
).
This means that we can use a seeded Engine in our unit tests, to make sure we always get the same results.
$random = new Randomizer(new Mt19937(123));
$builder = new SubDomainBuilder($random);
echo $builder->createsubDomain('example.com') . "\n";
Creating a random string before PHP 8.2
If you are on PHP 8.2 or higher you should use the Randomizer
. However, if you’re stuck on an earlier version of PHP, and want to generate a random
string, you can create your own Random generateor which uses the random_int
function as follows to generate your own random strings.
The class would roughly look as follows. You could even define often used key spaces in this class to re-use.
class RandomGenerator
{
public function getBytesFromString(string $keySpace, int $length): string {
$keySpaceLength = strlen($keySpace);
$randomString = '';
for ($i = 0; $i < $length; $i++) {
$randomString .= $keySpace[random_int(0, $keySpaceLength - 1)];
}
return $randomString;
}
}
Conclusion
In newer PHP versions is has become surprisingly easy to generate random strings. If youre on version 8.2 or higher, the
randomizer is the way to go. If youre a bit earlier than that, you can still use the random_int
function to create safe random strings.
If you want to get notified of my next blog post, join the news letter